4 min to read
04. Texture
텍스처링
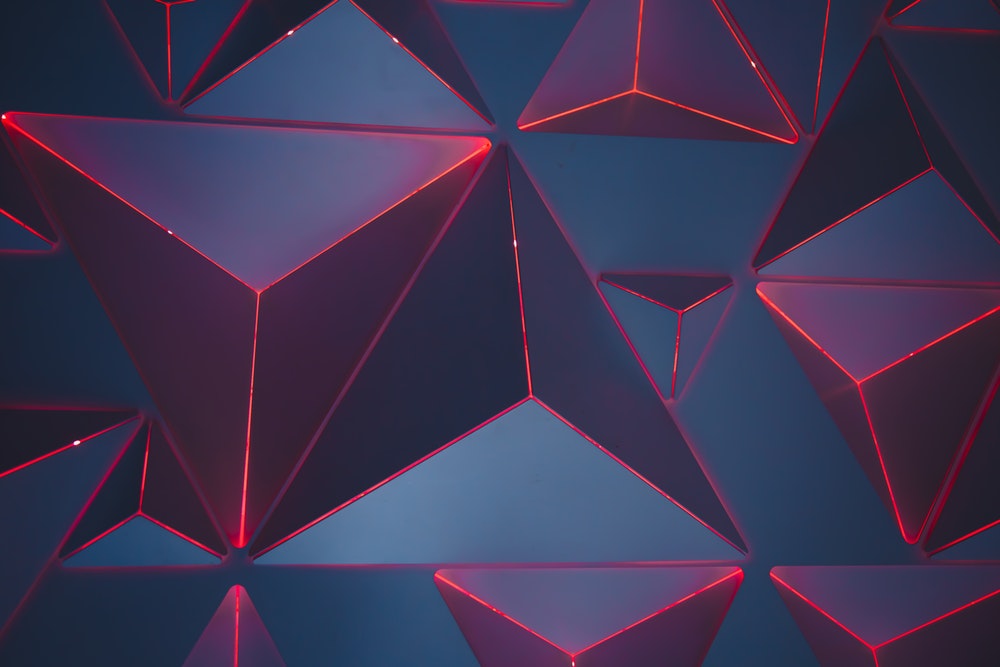
tip
사용하는 함수 : sampler2D(), tex2D(), lerp()
알아둬야할 개념 : uv좌표
Mesh에 텍스쳐링(텍스처를 붙이는 작업)하려면, 필요한 것이 UV 좌표계이랍니다.
각각의 정점은 위치의 제일 위에서 부터 2개의 float을 가질 수 있어요. 바로 U와 V죠. 이 좌표들은 텍스처에 접근할때 필요한데, 한번 보시죠!
삼각형에서 텍스처를 씌우면, 어떻게 왜곡되는지 살펴보세요.
Step 1: 텍스쳐 맵핑
struct VertexInput{
float4 vertex : POSITION;
float2 uv : TEXCOORD;
}
struct FragmentInput{
float4 vertex : POSITION;
float2 uv : TEXCOORD;
}
void vertex(VertexInput i){
FragmentInput o;
o.vertex = i.vertex;
o.uv = i.uv;
}
fixed4 fragment(FragementInput o){
return fixed4(1,1,1,1);
}
Step : UV좌표 움직이기
void surf (Input IN, inout SurfaceOutput o) {
fixed4 a = tex2D (_MainTex, IN.uv_MainTex.xy + _Time.xx * 0.5f);
fixed4 b = tex2D (_MainTex2, IN.uv_MainTex2.x + 0.5);
fixed4 result = lerp(a,b,0.5);
o.Emission = result;
o.Alpha = c.a;
}
Step : 텍스쳐 혼합
sampler2D _MainTex;
sampler2D _MainTex2;
struct Input{
float2 uv_MainTex;
float2 uv_MainTex2;
}
void surf (Input IN, inout SurfaceOutput o) {
fixed4 a = tex2D (_MainTex, IN.uv_MainTex);
fixed4 b = tex2D (_MainTex2, IN.uv_MainTex2);
fixed4 result = lerp(a,b,0.5);
o.Emission = result;
o.Alpha = c.a;
}
Step : 텍스쳐 회전
Shader "TextureRotate" {
Properties {
[HDR]_Color ("Color", Color) = (1,1,1,1)
_MainTex ("Albedo (RGB)", 2D) = "white" {}
_RotationSpeed("_RotationSpeed (텍스처 회전속도)", Range(0, 10)) = 1
}
SubShader {
Tags { "RenderType"="Transparent" "Queue" = "Transparent"}
LOD 200
CGPROGRAM
#pragma surface surf Lambert alpha:fade vertex:vert
#pragma target 2.0
sampler2D _MainTex;
struct Input {
fixed2 uv_MainTex;
};
fixed4 _Color;
fixed _ViewAmount; //보이는 정도
float _RotationSpeed; //텍스처 회전 속도
void vert(inout appdata_base v) {
float2 pivot = float2(0.5, 0.5); //텍스처 전체의 피봇을 0.5만큼 더함.
//Angle 반지름이 1인 원에서 삼각함수를 이용하여 (x,y) 좌표를 구할줄 알면 밑에 코드를 이해 할수 있다
float sinAngle = sin(_RotationSpeed);
float cosAngle = cos(_RotationSpeed);
float2x2 rotationMatrix = float2x2(cosAngle, -sinAngle, sinAngle, cosAngle);
v.texcoord.xy = mul(rotationMatrix, v.texcoord.xy - pivot) + pivot; //피봇 값을 빼고 더한이유는 텍스쳐는 0,0.좌표가 왼쪽하단이거나, 왼쪽상단이다, 중심은 (0.5, 0.5)이고 회전할때는 0,0 좌표 중심으로 회전시키기 때문에 텍스쳐 좌표를 피벗값을 마이너스 하여 중심을 0,0으로 맞추어 회전 시킨다음 다시 피봇값을 더 한다
}
////https://forum.unity.com/threads/rotation-of-texture-uvs-directly-from-a-shader.150482/
void surf (Input IN, inout SurfaceOutput o) {
fixed4 c = tex2D (_MainTex, IN.uv_MainTex) * _Color;
o.Emission = c;
o.Alpha = c.a;
}
ENDCG
}
}
Comments